Pricing API
A Price API is an interface that retrieves discounted prices for product variants based on promotions in the Chord OMS (Order Management System). It enables the front end to display real-time prices from the Chord OMS instead of static prices from the Chord CMS (Content Management System). You can create and manage promotions in the Chord OMS, which are reflected instantly on the front end.
The Price API evaluates specific types of promotions, automatically applying the highest discount to a product variant. Other types of promotions, such as coupon codes, are ignored. Prices on the front end may differ from checkout, but the OMS always selects the best promotion for lower prices during checkout.
The pricing API returns the discounted price of a variant based on the configured promotions in the Chord OMS. This allows the front end to display dynamic prices from the OMS instead of static prices from the CMS.
A typical use case would be a site-wide promotion (ex., 10% off on everything for Black Friday). An operator can create the promotion in the OMS, and the front end will reflect prices according to it without updating product content in the CMS.
For performance and simplicity reasons, the pricing API only supports specific promotions: only promotions that are automatically applied (i.e., no coupon code) with either no rule or with the following rules are evaluated by the API:
- Product(s)
- Variant(s)
- Store
- Order Contains a Subscription
- Subscription creation order
- Subscription interval greater
- Single Order (No Subscription Associated)
If multiple promotions match the criteria (sku and interval), the API returns the price for the βbestβ promotion (ie, the highest discount).
The API ignores all other promotions (coupon codes based on email, role, etc). Technically, prices could be different during checkout since all promotions are evaluated during checking. However, since the Chord OMS ALWAYS selects the best promotion for the order, prices should always be lower in checkout than shown on the product detail page (PDP)and/or the product listing page (PLP).
The pricing API implements a cache to deliver discount pricing information quickly for rendering on a product page. Prices are cached using the following criteria:
- Variant
- Quantity
- Store
- Interval length (optional)
- Interval units (optional)
There is no set expiration on cache lifetime. Once a discount price is calculated and cached, it will be retained indefinitely. Modifying a product's or variant's price or other attributes will not bust the cache.
The cache is busted (invalidated) in two circumstances:
- A promotion created, modified, or removed
- A price for a product or variant is created or modified
When any of the above actions occurs, the entire pricing cache is invalidated and will be rebuilt upon customer request.
GET /api/variants/{id|sku}/discount_prices
quantity (optional): The quantity that will be added to cart. Defaults to 1 interval_length (optional): The subscription length interval_units (optional): The subscription units (`day`, `week`, `month`, `year`).
ο»Ώ
You can find the document for the API here.
Chord OMS --> Promotions --> New Promotion:
Configure a Promotion that Apply to all orders, and provide 20% of per line item. Do not add any rules.

Configure another Promotion that Apply to all orders, and provide 50% off on Small Alstroemeria (alstroemeria-small) - Select Variants in Rules dropdown.

A request for alstroemeria-small will return the following (50% off):
A request for carnations-medium will return the following (20% off):
Configured in the Chord OMS: a 30% off on subscriptions with a 6 months interval
ο»Ώ
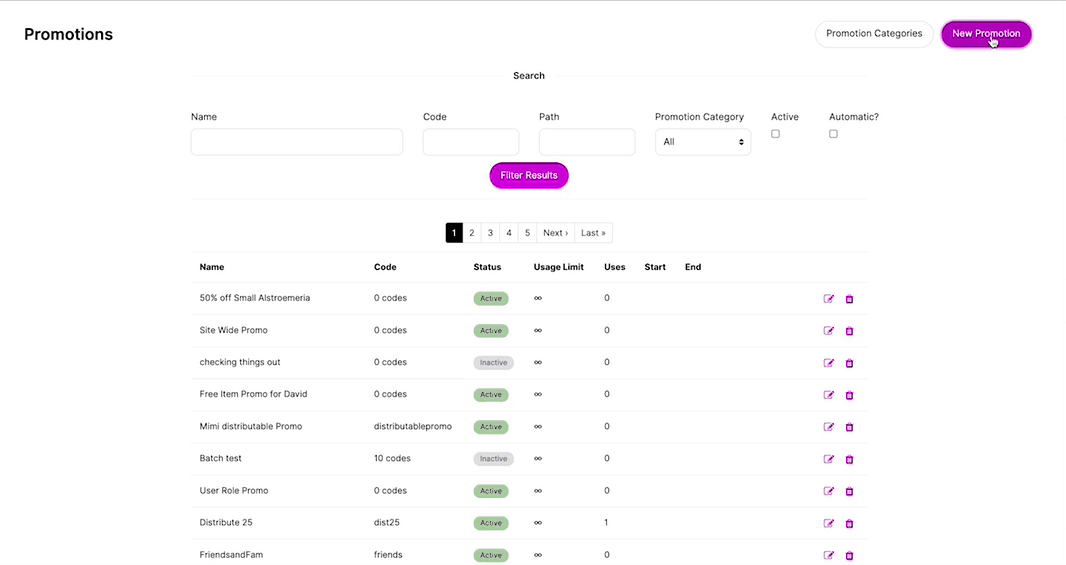
and a. 5% off on subscriptions with a 1 year interval

A request for a subscription to carnations-medium with a 6 months interval will return the following:
A request for a subscription to carnations-medium with a 1 year interval will return the following:
A request for a one-off purchase for carnations-medium will return the following (no discount):
The frontend can use the useVariantPrice hook (included in the nextjs-starter-autonomy package) to fetch the discounted price from the Chord OMS. The hook hides the implementation details (API call, formatting, caching, etc) making the integration seamless from a frontend point of view.
The useVariantPrice hook returns the discounted price of a variant based on the configured promotions in the Chord OMS. If the variant is not discounted, it returns the regular price.
Arguments:
Argument | Type | Description |
---|---|---|
input | VariantPriceInput | This contains the parameters for the API call: sku: string: The variant sku quantity?: number The quantity that will be added to cart (useful for tier-based promotions) intervalLength?: number: The interval length. Required to fetch the price of a subscription. intervalUnits?: string: The interval units (day, week, month, year). Required to fetch the price of a subscription. |
price (optional) | number | If present, the function will return this as the variantPrice in case of an API error. Typically, set as the CMS price. |
regularPrice (optional) | number | If present, the function will return this as the regularVariantPrice in case of an API error. Typically, set as the CMS regularPrice. |
intervalLength?: number: The interval length. Required to fetch the price of a subscription. | ||
regularPrice (optional) | ο»Ώ | ο»Ώ |
Returns:
Promise: A Promise that resolves when the discount price has been returned.
Attributes | Type | Description |
---|---|---|
variantPrice | number | The discounted variant price returned by the OMS. |
regularVariantPrice | number | The regular variant price returned by the OMS |
formattedVariantPrice | String | The formatted variant price according to the current locale. Ex. $8 |
formattedRegularVariantPrice | String | The formatted regular variant price according to the current locale. Ex. $8 |
isFetchingPrice | boolean | A flag indicating if the request has completed. |
Example:
ο»Ώ
π‘ For existing frontend running on an old version of the nextjs-starter-autonomy, it might be impossible to integrate the useVariantPrice hook. An alternative is to use the lower level getPrice function from the useProduct hook available in the react-autonomy package version >= 2.8.1
The getPrice function returns the price (including discount if applicable) for a product's variant by providing a SKU. The above use-variant-price hook could be implemented like this: